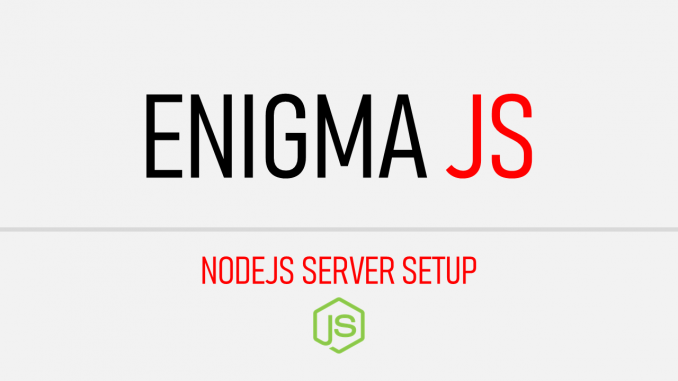
This post is part of the Enigma.js example series. Prior to the server setup, please complete the Enigma.js Applicaiton-ReactJS-Client-Setup.
It assumes that the node JS is already installed. If not, please download the nodejs. I will use windows 10 OS.
Set up folder
Create a folder server in the main app folder.
Install dependencies
For now, we will install the following dependencies. Since we have no database need at this point, we do not install any. But incase a need arises, we will use mongoDB with mongoose.
- cors
- express
Go to the command prompt and get to the app folder and run the following command
Once the node JS dependedncy installed, run the following command to install the enigma.js and ws (websocket) modules.
Set up Server
The code in server is typically split into
- Controllers
- Models
- Routes
Since, we do not have any need to persist or retrieve any entity from database, we start with setting up of Controllers and Routes at this point. However, we create the following folder structure inside the server
Set up Controllers
We create one controller to act on each components in qlik sense environment. To begin with, wee create a controller for the engine level data and call it qengine.ctrl.js and place the following code. We will get into the details of enigma.js later in the series. But for now, the focus is in getting basic code structure for the server module.
/**
* Get All Apps
*/
getApps: (req, res, next) => {
res.json({msg:'Gets all the apps from the qlik server'}));
}
}
Set up Routes
Similar to the controller, I create one route file that handles requests on each component of qlik sense. To begin with, I create a route file, qengine.js under routes folder and place the following code inside
module.exports = (router) => {
/**
* get All Apps
*/
router
.route('/apps')
.get(enginecontroller.getApps)
}
Create Express JS instance to bundle all routes
Create a file, index.js under routes folder and place the below code
module.exports = (router) => {
app(router)
qengine(router)
}
Create server entry point
Create a file app.js under the server folder and place the below code
const express = require("express")
const routes = require('./routes/')
const cors = require('cors')
const app = express()
const router = express.Router()
let port = 5000 || process.env.PORT
/** set up routes {API Endpoints} */
routes(router)
/** set up middlewares */
app.use(cors())
app.use('/api', router)
/** start server */
app.listen(port, () => {
console.log('Server started at port: ${port}');
});
Test the server setup
In the command prompt, go to the server folder and run the below command
You get the below output which means the server has started successful in the given port. Now access the below url in the browser
You must get the below output, which means that the server structure is complete and we are good to advance into creating working enigma.js application using Node js and React js.
Our folder structure looks like below.
– app(router) in routers does not exist
– on all places arrow function => is replaced by =>
– instead, backticks here are normal single quotes console.log(‘Server started at port: ${port}’) so you can’t see the port
Hi,
Thanks for the tutorial. As mentioned earlier you should replace => with => instead and remove the ; at the end as well. Also in the index file comment out the app(router) for now.
Thank you for the comment. I thought i fixed it. Going forth, I will use the default code block.
Hi everyone, I was searching for some tutorial about enigmajs. Thanks for the effort. For anyone searching how to make this part of tutorial, you will need to copy few extra files from the gitHub repo: https://github.com/CodeAtRoost/codewander-qlik-master-master/tree/master/server
./routes/app.js
./controllers/app.ctrl.js
Would be great to update it! Thanks again