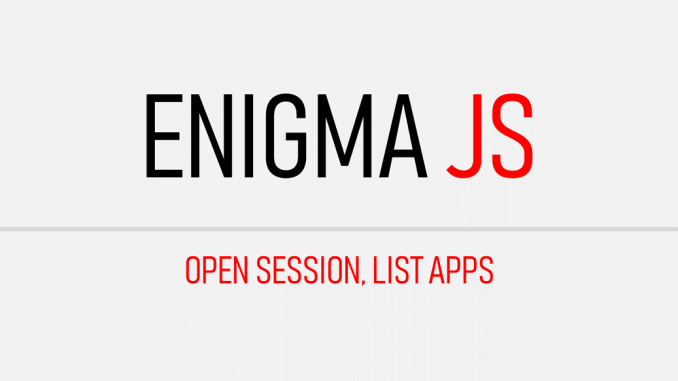
This post, enigma.js create and open session, is part of the Enigma.js example series. Prior to this post, please complete the ReactJS – Client setup and NodeJS – Server setup.
The enigma.js lets users access engine API of qlik sense. It basically is a wrapper to the websocket calls that sends and receives data from Qlik server. So, as the first step, we open a websocket session with Qlik server.
In our app, we created a qengine.ctrl.js controller under the controllers folder and qengine.js route file under the routes folder. The code that establishes connection to the qlik server will be placed in the controller file.
Load the dependencies
Load the dependencies, enigma.js and ws using the require call.
Load the schema which we will use in communication with the server. There are various versions of server available. I have used 12.67.2.json. When I used 3.1 version, I had trouble using one of the Engine API functions.
Place the below code in the beginning of the qengine.ctrl.js
const WebSocket = require('ws');
const schema = require('enigma.js/schemas/12.67.2.json');
var qixGlobal=null;
var qixSession=null
Set configuration to connect to engine
Declare the variable config that sets the details of the connection we will make. Place the below code
schema,
url: 'ws://localhost:4848/app/engineData',
createSocket: url => new WebSocket(url)
};
The url points to the app engineData, which is used to open and access engine data. In order to open sessions for each app, you have to use the respective app id.
This is the basic connection configuration required. For more information, please refer to enigma.js config definition.
Enigma.js Create and Open a Session
Now use the configuration above and create a websocket session. We will also by default, get all the apps in the server. Place the below code in the qengine.ctrl.js file inside the getApps call. We will return the resulting json as response to the API
qixSession.open().then((global) => {
qixGlobal=global;
qixGlobal.getDocList().then(function(list){res.json({msg:list})});
});
As you can see in the above code, getDocList is used to retrieve the list of apps.
Test the script
Now, restart the server. Type the below url to get the json response of all the apps in the qlik server. Make sure that you have the Qlik server that you target is up and running.
You will get the list of apps as json. You can use the app id retrieved from this call to connect to individual apps.
we can also use the same structure to list all the streams.
Please find the complete code of qengine.ctrl.js.
const enigma = require('enigma.js');
const WebSocket = require('ws');
const schema = require('enigma.js/schemas/12.67.2.json');
var qixGlobal=null;
var qixSession=null
const qix_config = {
schema,
url: 'ws://localhost:4848/app/engineData',
createSocket: url => new WebSocket(url)
};
module.exports = {
/**
* Get All Apps
*/
getApps: (req, res, next) => {
qixSession=enigma.create(qix_config);
qixSession.open().then((global) => {
qixGlobal=global;
qixGlobal.getDocList().then(function(list){res.json({msg:list})});
});
}
}
– url ws://localhost:4848/app/engineData does not exist, you did not explain what is running on this port and how to set up it
– missing yarn add enigma.js ws or npm i –save enigma.js ws
– var should be let
maybe use url wss://playground.qlik.com/app/engineData or show user how to install localhost engine