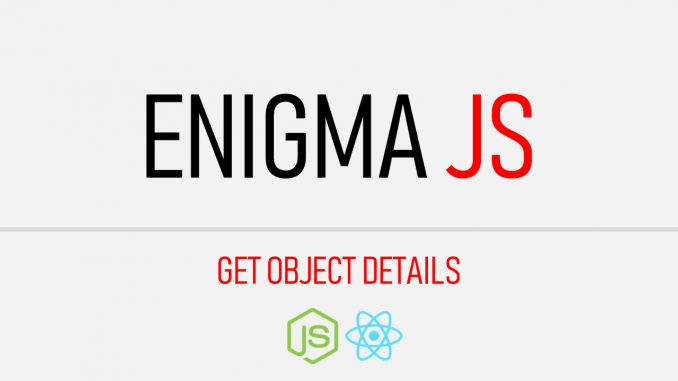
This post, Qlik sense enigma js example – Get Definition of App Objects, is part of the Qlik sense Enigma.js Example Series. This post will explain how to get the definition and other details of a given object.
Prior to this post, please complete the following posts:
- ReactJS – Client setup,
- NodeJS – Server setup
- Qlik sense Enigmajs Create, Open session and list apps
- Qlik sense enigma.js – example – Display Apps using ReactJS
- Qlik sense enigma js example – Get App Objects List
The above posts will help you get the basic setup, list all the apps in the qlik sense server, open a desired app and list all the master measures, dimensions, visualization, variables and sheets.
The latest working app can be found below
Download CodeWander Enigma JS Example
Demo
Steps in getting the details of a master measure
Set up routes
On the server, in ./routes/app.js introduce 2 new routes
- First to get the master measure detail from the current app
- Second to get the master measure detail from an app with app a given app id
* return details of the master measure
*/
router
.route('/app/current/measuredetails/:measure_id')
.get(appcontroller.getMeasureDetails)
/**
* open an App and return details of a master measure
*/
router
.route('/app/:app_id/measuredetails/:measure_id')
.get(appcontroller.getMeasureDetails)
Fetch the master measure details
The details of a master measure can be retrieved using the getMeasure and getLayout function.
On the server, in ./controllers/app.ctrl.js of server folder, we introduce a new function, getMeasureDetails
var measure_id=app_id=req.params.measure_id
if (req.params.app_id!=null)
{
app_id=req.params.app_id
var config={
schema,
url: server_url+app_id,
createSocket: url => new WebSocket(url)
};
if (qSession!=null)qSession.close();
qSession=enigma.create(config);
qSession.open().then((global) => {
qGlobal=global;
qGlobal.openDoc(app_id).then((app) => {
currApp=app;
currApp.getMeasure(measure_id).then(function(qObject){
qObject.getLayout().then(function(qProp){
res.json({msg:qProp})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)}) ;
})
},(err)=>{ console.log(err);res.json(err)})
}
else{
currApp.getMeasure(measure_id).then(function(qObject){
//console.log("created object");
qObject.getLayout().then(function(qProp){
res.json({msg:qProp})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)})
}
}
Setup Routes and Fetch Details of Dimensions, Master Visualizations and Sheets
Using the method illustrated above for Master Measures, the routes are setup for dimensions and other components. For Dimensions, GetDimension function of enigma.js is used. For Sheets and Master Visualizations, GetObject function of enigma.js is used.
Setup Routes in ./routes/app.js of server folder
Routes for Dimension
* return details of the master dimension
*/
router
.route('/app/current/dimensiondetails/:dimension_id')
.get(appcontroller.getDimensionDetails)
/**
* open an App and return details of a master dimension
*/
router
.route('/app/:app_id/dimensiondetails/:dimension_id')
.get(appcontroller.getDimensionDetails)
Routes for Sheets and Master Visualizations
* return details of the object
*/
router
.route('/app/current/objectdetails/:object_id')
.get(appcontroller.getObjectDetails)
/**
* open an App and return details of an object
*/
router
.route('/app/:app_id/objectdetails/:object_id')
.get(appcontroller.getObjectDetails)
Functions to fetch details in ./controllers/app.ctrl.js
Fetch Master Measure Details
var measure_id=app_id=req.params.measure_id
if (req.params.app_id!=null)
{
app_id=req.params.app_id
var config={
schema,
url: server_url+app_id,
createSocket: url => new WebSocket(url)
};
if (qSession!=null)qSession.close();
qSession=enigma.create(config);
qSession.open().then((global) => {
qGlobal=global;
qGlobal.openDoc(app_id).then((app) => {
currApp=app;
currApp.getMeasure(measure_id).then(function(qObject){
qObject.getLayout().then(function(qProp){
res.json({msg:qProp})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)}) ;
})
},(err)=>{ console.log(err);res.json(err)})
}
else{
currApp.getMeasure(measure_id).then(function(qObject){
//console.log("created object");
qObject.getLayout().then(function(qProp){
res.json({msg:qProp})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)})
}
}
Fetch Sheets and Master Visualization Details
var object_id=app_id=req.params.object_id
if (req.params.app_id!=null)
{
app_id=req.params.app_id
var config={
schema,
url: server_url+app_id,
createSocket: url => new WebSocket(url)
};
if (qSession!=null)qSession.close();
qSession=enigma.create(config);
qSession.open().then((global) => {
qGlobal=global;
qGlobal.openDoc(app_id).then((app) => {
currApp=app;
currApp.getObject(object_id).then(function(qObject){
qObject.getLayout().then(function(qProp){
res.json({msg:qProp})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)}) ;
})
},(err)=>{ console.log(err);res.json(err)})
}
else{
currApp.getObject(object_id).then(function(qObject){
//console.log("created object");
qObject.getLayout().then(function(qProp){
res.json({msg:qProp})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)})
}
}
Test the Script
Restart the sever by typing the following command in the command prompt inside the server folder
node app.js
Go to the browser and launch the below urls and you should the output similar to the one below
http://localhost:5000/api/app/Helpdesk%20Management%20App2.qvf/measuredetails/CqgPvhm
You should get the following json object
Display Measure Details in ReactJS Application Client
Unitl now, the ReactJS Client has one single component QMeasures to display the master measures details fetched using getMeasures api of the server. Now we will introduce another component QMeasure which the QMeasures will call for each measures in the list. The QMeasure component will fetch the additional details of the measure.
The QMeasure component will display the definition of the measure. But it can be used to display any details from the response JSON shown above.
Call QMeasure from QMeasures (.js) Component
return (<QMeasure ref={qMeasure.qInfo.qId} qMeasure={qMeasure} />);
})
Fetch Measure Details in QMeasure(.js) Component using AJAX Call
{
let self=this;
//this.props.getMeasureDetails(_id)
axios.get(`${qlik_server}app/current/measuredetails/${_id}`)
.then((res) => {
let qMeasureDetails = res.data.msg;
self.props.qMeasure.qMeasureDetails = qMeasureDetails;
self.forceUpdate()
//self.render()
}).catch((err) => {
console.log(err);
//self.render();
return {"error": "An error occurred"}
})
}
Display Fetched Details in QMeasure Component
let self=this;
self.getDetails(this.props.qMeasure.qInfo.qId)
const qDetails=this.props.qMeasure.qMeasureDetails==null? "Fetching": this.props.qMeasure.qMeasureDetails.qMeasure.qDef;
console.log(qDetails);
return (
<tr>
<th scope="row" >{this.props.qMeasure.qMeta.title}</th>
<td>{this.props.qMeasure.qMeta.description}</td>
<td>{qDetails}</td>
<td><button type="button" className="btn btn-outline-primary" onClick={(e) => self.getDetails(this.props.qMeasure.qInfo.qId)}>Details</button></td>
</tr>
);
}
Fetch and Display Details of Master Dimension, Sheets and Master Visualization
Using the method illustrated above, the details of Sheets, Master Dimensions and Master Visualizations are fetched.
The new components introduced for this purpose are
- QSheet – called from QSheets
- QVisualization – called from QVisualizations
- QDimension – called from QDimenions
Test the script
Restart ReactJS Client using the following command in the command prompt npm start localhost:3000 to launch the app Click on Apps to see all the apps Click on an app to see basic app deteails and Open App button Now Click on Sheets, Measures, Dimensions, Master Visualization and Variables to see all the objects
Leave a Reply