This post, Qlik sense enigma js example using node js and react – Create Sheet, is part of theĀ Qlik sense Enigma.js Example Series. This post will explain how to create a new sheet using enigma js.
Prior to this post, please complete the following posts:
- ReactJS – Client setup,
- NodeJS – Server setup
- Create, Open session and list apps
- Display Apps using ReactJS
- Get App Objects List
- Get Definition of App Objects
- Create New App
The above posts will help you get the basic setup, list all the apps in the qlik sense server, open a desired app and list all the master measures, dimensions, visualization, variables and sheets and also to create new app.
The latest working app can be found below
Download CodeWander Enigma JS Example
Enigma JS Example Create Sheet
There are two parts in creating the new sheet. First the server side api that actually interacts with the qlik engine and the client part for user interface. This post (part 1) covers the server side and the next post will cover the client side programming using react js.
Server Side Programming
There are two changes on the server side
- Create routes for the client to consume
- Write a function createSheet that gets the input and generate a new sheet in a given app
Create routes for the client to consume
In the file .\server\routes\app.js, urls are created to give create a sheet with a name and optionally a description, in the current app or another app.
/**
* create sheet with name and desc in the current app
*/
router
.route('/app/current/create/sheet/:name/desc/:desc')
.get(appcontroller.createSheet)
/**
* open an app and create sheet with name and desc in the app
*/
router
.route('/app/:app_id/create/sheet/:name/desc/:desc')
.get(appcontroller.createSheet)
/**
* create sheet with name and no desc in the current app
*/
router
.route('/app/current/create/sheet/:name')
.get(appcontroller.createSheet)
/**
* open an app and create sheet with name and no desc in the app
*/
router
.route('/app/:app_id/create/sheet/:name')
.get(appcontroller.createSheet)
Write a function to create a sheet with name and description
In the file, server/controllers/app.ctrl.js, write the function createSheet. The qParamsSheet variable is the one that defines the sheet. You can extend the function to get thumbnail and other details also.
The response is given in specific json format. This new format ensures that a response is received by the client even in the event of failure. This has been missing in earlier functions that I wrote. Below is the format
{"qRequest":"createSheet", "qResponseStatus": "", "qResponseMsg": ""}
Here is the complete function.
createSheet: (req,res,next)=>{
var sheetName = decodeURIComponent(req.params.name);
var sheetDesc = req.params.desc == null ? "": decodeURIComponent(req.params.desc);
var qParamsSheet={"qInfo":{"qType":"sheet"},
"qExtendsId":"",
"qStateName":"$",
"qMetaDef":{"title":sheetName,"description":sheetDesc},
"rank":0,
"thumbnail":{"qStaticContentUrlDef":null},
"columns":24,
"rows":12,
"cells":[],
"qChildListDef":{"qData":{"title":"/title"}}}
//console.log(JSON.stringify(qParamsSheet));
if (req.params.app_id!=null)
{
app_id=req.params.app_id
var config={
schema,
url: server_url +app_id,
createSocket: url => new WebSocket(url)
};
if (qSession!=null)qSession.close();
qSession=enigma.create(config);
qSession.open().then((global) => {
qGlobal=global;
qGlobal.openDoc(app_id).then((app) => {
currApp=app;
currApp.createObject(qParamsSheet).then(function(qObject){
qObject.getLayout().then(function(qProp){
res.json({"qRequest":"createSheet","qResponseStatus": "success" , "qResponseMsg":qProp})
},
(e)=> {console.log(err);res.json({"qRequest":"createSheet", "qResponseStatus": "error", "qResponseMsg": null})});
}
,(err)=>{ console.log(err);res.json({"qRequest":"createSheet","qResponseStatus": "success" , "qResponseMsg":null})}) ;
})
},(err)=>{ console.log(err);res.json({"qRequest":"createSheet","qResponseStatus": "success" , "qResponseMsg":null})})
}
else{
currApp.createObject(qParamsSheet).then(function(qObject){
//console.log("created object");
qObject.getLayout().then(function(qProp){
res.json({"qResponseStatus": "success" , "qResponseMsg":qProp})
},
(e)=> {console.log(err);res.json({"qRequest":"createSheet","qResponseStatus": "success" , "qResponseMsg":null})});
}
,(err)=>{ console.log(err);res.json({"qRequest":"createSheet","qResponseStatus": "success" , "qResponseMsg":null})}) ;
}
}
Testing the API – Successful Response
Now restart the server using node app.js command and send the below request from the browser and you will get a successful message as shown
Request:
http://localhost:5000/api/apps/create/Test App via Enigma
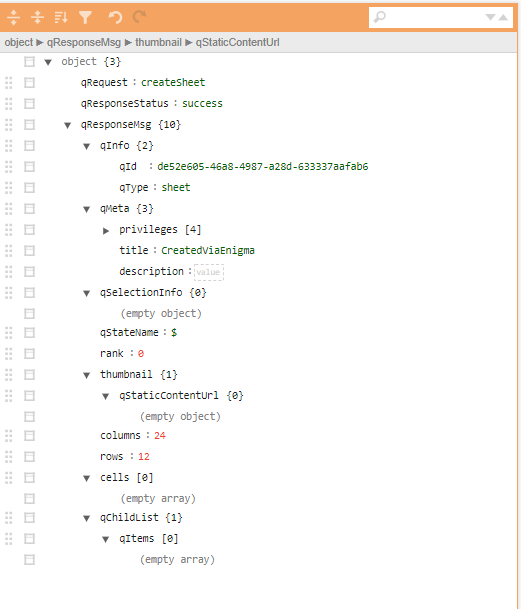
This concludes server side programming to create sheet in a qlik sense app using enigma js
Leave a Reply