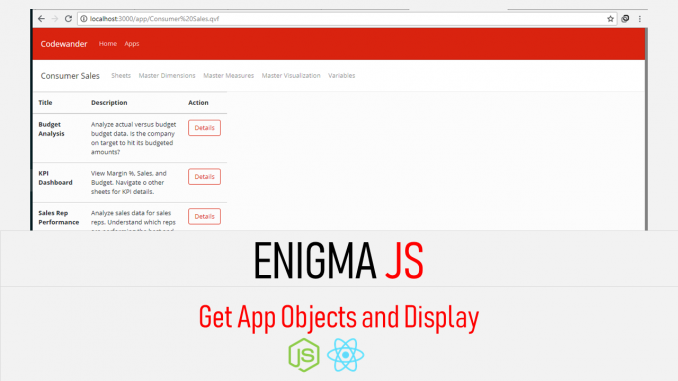
This post, Qlik sense enigma js example – Get App Objects List, is part of the Qlik sense Enigma.js Example Series. Prior to this post, please complete the following posts:
- ReactJS – Client setup,
- NodeJS – Server setup
- Qlik sense Enigmajs Create, Open session and list apps
- Qlik sense enigma.js – example – Display Apps using ReactJS
The above posts will help you get the basic setup, list all the apps in the qlik sense server and open a desired app.
The latest working app can be found below
Download CodeWander Enigma JS Example
This post will explain the methods used to get the following details of the app.
-
- Sheets
- Master Dimensions
- Master Measures
- Master Visualizations
- Variables
Demo
Steps in getting the list of measures, dimensions, master visualizations and variables in an app
-
- Create a session object list (wee shall consider master dimensions for this example)
- Get Layout of the list
Create a session object of the list of dimensions
The enigma.js function createSessionObject is used to create a list object. The definition of what the list object should contain is defined by a json structure and is passed as a parameter. This json structure varies depends on what you want to fetch. For this example, we will create a list to fetch dimensions. The definition is as below.
"qInfo": {
"qType": "DimensionList",
"qId": ""
},
"qDimensionListDef": {
"qType": "dimension",
"qData": {
"title": "/qMetaDef/title",
"tags": "/qMetaDef/tags",
"grouping": "/qDim/qGrouping",
"info": "/qDimInfos"
}
}
}
You can find the definitions (parameters) for createSessionObject function to fetch various lists below
Get Layout of the list
This is the step where we fetch list of objects (in this example, dimensions). The response will have the list of dimensions and its basic details. The enigma.js function getLayout is used to achieve this. The overall function call looks like this. qParam is the json structure we discussed above. qProp is the response that contains the list of dimensions
.then(function(qObject){
console.log("created object");
qObject.getLayout()
.then(function(qProp){
res.json({msg:qProp.qDimensionList.qItems})
}, (e)=> {console.log(err);res.json(err)}); }
Steps in getting the list of sheets in an app
The getObjects function of enigma.js can be used to get the list of sheets and master visualizations in an app. The following is the json structure that has to be passed for sheets and master visualizations.
"qOptions": {
"qTypes": [
"sheet"
],
"qIncludeSessionObjects": false,
"qData": {}
}
}
currApp.getObjects(qOptionsSheet)
.then(function(qProp){
res.json({msg:qProp})}
,(err)=>{ console.log(err);res.json(err)}
)
Note: You can also use the CreateSessionObject and GetLayout functions to get the list of sheets as well.
Complete Implementation
In our app, we implement these functions in the app.ctrl.js to fetch the dimensions, measures and variables. Change the app.ctrl.js file as below.
var currApp=null;
var qGlobal=null;
var qSession=null
const enigma = require('enigma.js');
const WebSocket = require('ws');
const schema = require('enigma.js/schemas/12.67.2.json');
module.exports = {
/**
* get App by Id
*/
getApp: (req, res, next) => {
var qOptionsSheet={
"qOptions": {
"qTypes": [
"sheet"
],
"qIncludeSessionObjects": false,
"qData": {}
}
}
var app_id=decodeURIComponent(req.params.app_id);
var config={
schema,
url: 'ws://localhost:4848/app/'+app_id,
createSocket: url => new WebSocket(url)
};
qSession=enigma.create(config);
qSession.open().then((global) => {
// global === QIX global interface
qGlobal=global;
qGlobal.openDoc(app_id).then((app) => {
currApp=app;
app.getAppProperties().then(function(qProp){res.json({msg:qProp})}
,(err)=>{ console.log(err);res.json(err)}) ;
})
});
},
getSheets: (req,res,next)=>{
var qOptionsSheet={
"qOptions": {
"qTypes": [
"sheet"
],
"qIncludeSessionObjects": false,
"qData": {}
}
}
if (req.params.app_id!=null)
{
app_id=req.params.app_id
var config={
schema,
url: 'ws://localhost:4848/app/'+app_id,
createSocket: url => new WebSocket(url)
};
if (qSession!=null)qSession.close();
qSession=enigma.create(config);
qSession.open().then((global) => {
qGlobal=global;
qGlobal.openDoc(app_id).then((app) => {
currApp=app;
currApp.getObjects(qOptionsSheet).then(function(qProp){res.json({msg:qProp})}
,(err)=>{ console.log(err);res.json(err)}) ;
})
},(err)=>{ console.log(err);res.json(err)} )
}
else{
currApp.getObjects(qOptionsSheet).then(function(qProp){
console.log("Returing Data");
res.json({msg:qProp})
}
,(err)=>{ console.log(err);res.json(err)}) ;
}
}
,
getDimensions: (req,res,next)=>{
var qParam={"qInfo":{"qType":"DimensionList","qId":""},"qDimensionListDef":{"qType":"dimension","qData":{"title":"/qMetaDef/title","tags":"/qMetaDef/tags","grouping":"/qDim/qGrouping","info":"/qDimInfos"}}}
if (req.params.app_id!=null)
{
app_id=req.params.app_id
var config={
schema,
url: 'ws://localhost:4848/app/'+app_id,
createSocket: url => new WebSocket(url)
};
if (qSession!=null)qSession.close();
qSession=enigma.create(config);
qSession.open().then((global) => {
qGlobal=global;
qGlobal.openDoc(app_id).then((app) => {
currApp=app;
currApp.createSessionObject(qParam).then(function(qObject){
console.log("created object");
qObject.getLayout().then(function(qProp){
res.json({msg:qProp.qDimensionList.qItems})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)}) ;
})
},(err)=>{ console.log(err);res.json(err)})
}
else{
currApp.createSessionObject(qParam).then(function(qObject){
console.log("created object");
qObject.getLayout().then(function(qProp){
res.json({msg:qProp.qDimensionList.qItems})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)})
}
},
getMeasures: (req,res,next)=>{
var qParam={"qInfo":{"qType":"MeasureList","qId":""},"qMeasureListDef":{"qType":"measure","qData":{"title":"/qMetaDef/title","tags":"/qMetaDef/tags"}}}
if (req.params.app_id!=null)
{
app_id=req.params.app_id
var config={
schema,
url: 'ws://localhost:4848/app/'+app_id,
createSocket: url => new WebSocket(url)
};
if (qSession!=null)qSession.close();
qSession=enigma.create(config);
qSession.open().then((global) => {
qGlobal=global;
qGlobal.openDoc(app_id).then((app) => {
currApp=app;
currApp.createSessionObject(qParam).then(function(qObject){
console.log("created object");
qObject.getLayout().then(function(qProp){
res.json({msg:qProp.qMeasureList.qItems})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)}) ;
})
},(err)=>{ console.log(err);res.json(err)})
}
else{
currApp.createSessionObject(qParam).then(function(qObject){
console.log("created object");
qObject.getLayout().then(function(qProp){
res.json({msg:qProp.qMeasureList.qItems})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)})
}
}
,
getVariables: (req,res,next)=>{
var qParam={"qInfo":{"qType":"VariableList","qId":""},"qVariableListDef":{"qType":"variable","qData":{"tags":"/tags"}}}
if (req.params.app_id!=null)
{
app_id=req.params.app_id
var config={
schema,
url: 'ws://localhost:4848/app/'+app_id,
createSocket: url => new WebSocket(url)
};
if (qSession!=null)qSession.close();
qSession=enigma.create(config);
qSession.open().then((global) => {
qGlobal=global;
qGlobal.openDoc(app_id).then((app) => {
currApp=app;
currApp.createSessionObject(qParam).then(function(qObject){
//console.log("created object");
qObject.getLayout().then(function(qProp){
res.json({msg:qProp.qVariableList.qItems})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)}) ;
})
},(err)=>{ console.log(err);res.json(err)})
}
else{
currApp.createSessionObject(qParam).then(function(qObject){
//console.log("created object");
qObject.getLayout().then(function(qProp){
res.json({msg:qProp.qVariableList.qItems})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)})
}
}
,
getMasterVisualizations: (req,res,next)=>{
var qParam={"qInfo":{"qType":"masterobject","qId":""},"qAppObjectListDef":{"qType":"masterobject","qData":{"name":"/metadata/name","visualization":"/visualization","tags":"/metadata/tags"}}}
if (req.params.app_id!=null)
{
app_id=req.params.app_id
var config={
schema,
url: 'ws://localhost:4848/app/'+app_id,
createSocket: url => new WebSocket(url)
};
if (qSession!=null)qSession.close();
qSession=enigma.create(config);
qSession.open().then((global) => {
qGlobal=global;
qGlobal.openDoc(app_id).then((app) => {
currApp=app;
currApp.createSessionObject(qParam).then(function(qObject){
//console.log("created object");
qObject.getLayout().then(function(qProp){
res.json({msg:qProp.qAppObjectList.qItems})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)}) ;
})
},(err)=>{ console.log(err);res.json(err)})
}
else{
currApp.createSessionObject(qParam).then(function(qObject){
//console.log("created object");
qObject.getLayout().then(function(qProp){
res.json({msg:qProp.qAppObjectList.qItems})
},
(e)=> {console.log(err);res.json(err)});
}
,(err)=>{ console.log(err);res.json(err)})
}
}
}
We then expose these functions by providing appropriate urls in the router for our client app to consume. Change the \routes\app.js as below.
const enginecontroller = require('./../controllers/qengine.ctrl')
module.exports = (router) => {
/**
* open App and return sheets
*/
router
.route('/app/:app_id')
.get(appcontroller.getApp)
/**
* return sheets from current app
*/
router
.route('/app/current/sheets')
.get(appcontroller.getSheets)
/**
* open an App and return sheets
*/
router
.route('/app/:app_id/sheets')
.get(appcontroller.getSheets)
/**
* return dimensions from current app
*/
router
.route('/app/current/dimensions')
.get(appcontroller.getDimensions)
/**
* open an App and return dimensions
*/
router
.route('/app/:app_id/dimensions')
.get(appcontroller.getDimensions)
/**
* return measures from current app
*/
router
.route('/app/current/measures')
.get(appcontroller.getMeasures)
/**
* open an App and return measures
*/
router
.route('/app/:app_id/measures')
.get(appcontroller.getMeasures)
/**
* return variables from current app
*/
router
.route('/app/current/variables')
.get(appcontroller.getVariables)
/**
* open an App and return variables
*/
router
.route('/app/:app_id/variables')
.get(appcontroller.getVariables)
/**
* return master visualizations from current app
*/
router
.route('/app/current/master_visualizations')
.get(appcontroller.getMasterVisualizations)
/**
* open an App and return master visualizations
*/
router
.route('/app/:app_id/master_visualizations')
.get(appcontroller.getMasterVisualizations)
}
Test the script
Restart the sever by typing the following commad in the command prompt inside the server folder
node app.js
Go to the browser and launch the below urls and you should the output similar to the one below
http://localhost:5000/api/app/Helpdesk%20Management.qvf/dimensions http://localhost:5000/api/app/Helpdesk%20Management.qvf/measures http://localhost:5000/api/app/Helpdesk%20Management.qvf/variables http://localhost:5000/api/app/Helpdesk%20Management.qvf/sheets http://localhost:5000/api/app/Helpdesk%20Management.qvf/master_visualizations
Displaying App Objects List using React JS
New Components
Create new components, state objects and actions to
- Open an app
- List Sheets, Measurers, Dimensions, Master Visualizations and Variables
Components
- PageHeader.js: To navigate between list of apps
- QOpenAppButton.js: To open an app from the list
- qApp.js: The container app consisting of app header and other containers
- QDimensions.js, QMeasurers.js, QSheets.js, QVariables.js and QVisualizations.js: To display list of app objects
- Home.js: To display a landing page
State Objects in redux/reducers/qApps.js
- qSheets to store all the sheet objects
- qMasterMeasures to store all the measures
- qMasterDimensions to store all the dimensions
- qMasterVisualizations to store all the master visualizations
- qVariables to store all the variables
Actions in redux/actions/actions.js
- openApp
- getMeasures
- getDimensions
- getMasterVisualizations
- getVariables
Please download the latest working copy to update these new components
Download CodeWander Enigma JS Example
Test the script
Restart ReactJS Client using the following command in the command prompt npm start localhost:3000 to launch the app Click on Apps to see all the apps Click on an app to see basic app deteails and Open App button Now Click on Sheets, Measures, Dimensions, Master Visualization and Variables to see all the objects![]()
![]()
Leave a Reply